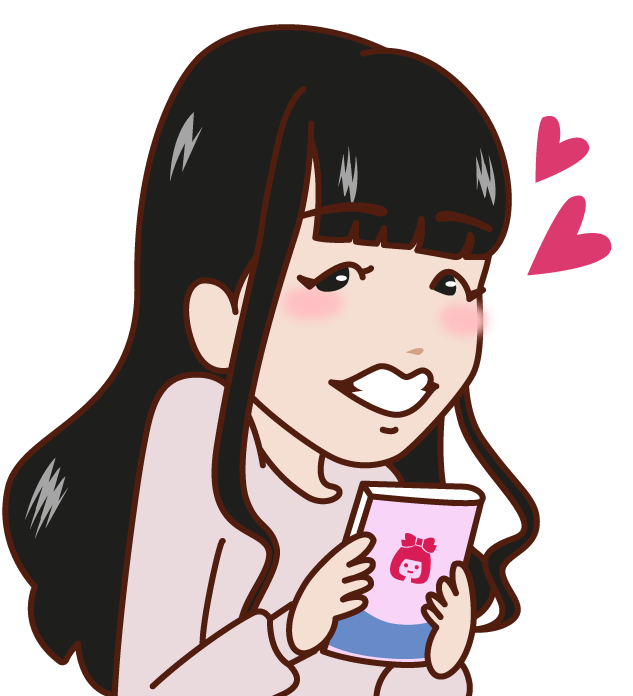
フロントエンド
エンジニア
カナちゃん
前回の記事から2年以上も経っておりました。。。
今回は、Perlを使ってMovable Typeのプラグインを作ってみました。
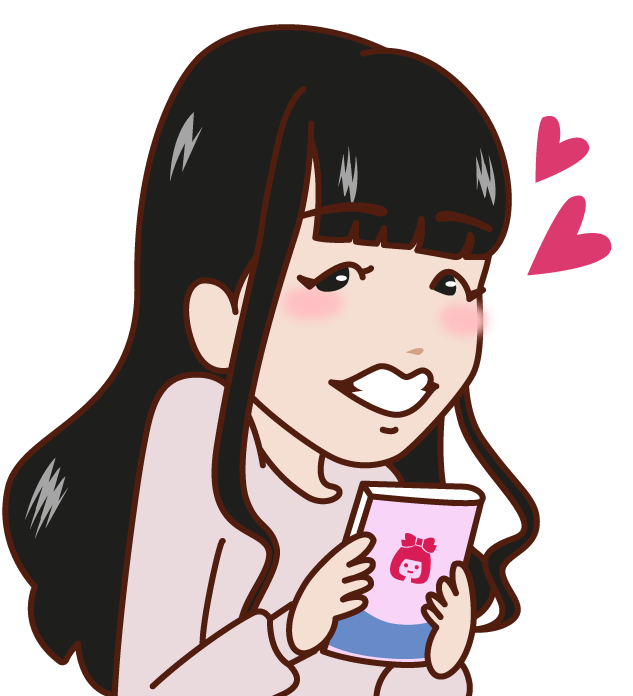
フロントエンド
エンジニア
カナちゃん
フロントエンドの技術系記事を書けとのお達しがあったのですが、フロントエンド要素が3ミリくらいになってしまいました。
目次
MTのプラグイン作成に必要な作業
いくつかファイルを作って、MTのpluginsフォルダにアップするだけです!
必要なファイルは今から作っていきます💪
基礎編:管理画面に固定のJSを読み込むようにする
管理画面のテンプレートを直接編集して追加するのではなく、
プラグインの機能として追加できるようにしてみたいと思います。
ディレクトリ構造
├── mt-static
│ └── plugins
│ └── sample
│ └── js
│ └── sample.js //管理画面に設置したいJS
└── plugins
└── sample
├── config.yaml
├── lib
│ └── sample
│ ├── CMS.pm
│ └── L10N
│ │ └── ja.pm
│ └── L10N.pm
└── tmpl
└── insert.tmpl
パスさえ合っていれば、JSファイルはどこに置いても大丈夫です!
基本設定
config.yaml
id: sample
name: <__trans phrase="sample L10N">
version: 1.0
description: <__trans phrase="_PLUGIN_DESCRIPTION">
author_name: <__trans phrase="_PLUGIN_AUTHOR">
author_link: https://logical-studio.com/
l10n_class: sample::L10N
callbacks:
MT::App::CMS::template_source.footer: $sample::sample::CMS::template_source_footer
name
description
author_name
後述のローカライズで指定するために変数にしていますが、
author_link
のように任意のテキストに変えても問題ないです。
l10n_class
後述のローカライズで使用します。
callbacks
コールバックとは、MTのコードの色々な場面に設置されているフックポイントを「フック」してMTを機能拡張します。 フックポイントはオブジェクトの編集や保存、ページ構築やAPIの操作といった場所に設置されています。
config.yamlでフックポイントと、それを処理するハンドラ関数を指定する事で、コールバックが利用可能になります。
https://github.com/movabletype/Documentation/wiki/Japanese-plugin-dev-3-2
今回はフッターのテンプレートに処理を追加したいので、template_source.footer
を指定しています。
ローカライズ
次は、プラグインを多言語化するために必要なファイルを作成していきます。
L10N.pm
package sample::L10N;
use strict;
use base 'MT::Plugin::L10N';
1;
辞書ファイルのひな形です🦁
これを元に多言語ファイルを作成します。
ja.pm
package sample::L10N::ja;
use strict;
use base 'sample::L10N';
use vars qw( %Lexicon );
%Lexicon = (
'sample L10N' => 'sampleプラグイン',
'_PLUGIN_DESCRIPTION' => '管理画面にJSを読み込むプラグインです',
'_PLUGIN_AUTHOR' => '株式会社ロジカルスタジオ',
);
1;
今回は日本語の辞書ファイルだけ設置します。
処理
CMS.pm
package sample::CMS;
use strict;
use warnings;
use MT::Util qw( trim );
sub template_source_footer {
my ( $cb, $app, $tmpl ) = @_;
my $plugin = MT->component('sample');
my $position = quotemeta(q{</body>});
my $plugin_tmpl = File::Spec->catdir( $plugin->path, 'tmpl', 'insert.tmpl' );
my $insert = qq{<mt:include name="$plugin_tmpl" component="sample">\n};
$$tmpl =~ s/($position)/$insert$1/;
}
1;
</body>
の直前にinsert.tmplを追加する処理を書いています。
insert.tmpl
<!-- Add sample.js -->
<script type="text/javascript" src="/cms/mt-static/plugins/sample/js/sample.js"></script>
<!-- Add sample.js -->
たったこれだけで、固定のJSを読み込むことができるようになりました!
応用編:管理画面からファイルのパスを変更できるようにする
読み込むJSファイルを変更したいとき、いちいちプラグインを書き換えるのが面倒臭かったので
管理画面から変更できるようにしてみます。
config.yaml
id: sample
name: <__trans phrase="sample L10N">
version: 2.0
description: <__trans phrase="_PLUGIN_DESCRIPTION">
author_name: <__trans phrase="_PLUGIN_AUTHOR">
author_link: https://logical-studio.com/
l10n_class: sample::L10N
system_config_template: system.tmpl
settings:
filepath:
scope: system
default: "/cms/mt-static/plugins/sample/js/sample.js"
callbacks:
MT::App::CMS::template_source.footer: $sample::sample::CMS::template_source_footer
変更点
- 3行目
- 9〜13行目
管理画面にプラグインの設定を追加するための記述を入れました。
ja.pm
package sample::L10N::ja;
use strict;
use base 'sample::L10N';
use vars qw( %Lexicon );
%Lexicon = (
'sample L10N' => 'sampleプラグイン',
'_PLUGIN_DESCRIPTION' => '管理画面にJSを読み込むプラグインです',
'_PLUGIN_AUTHOR' => '株式会社ロジカルスタジオ',
'FilePathLabel' => 'パス',
);
1;
変更点
- 11行目
入力フィールドのラベルを追加します。
CMS.pm
package sample::CMS;
use strict;
use warnings;
use MT::Util qw( trim );
sub template_source_footer {
my ( $cb, $app, $tmpl ) = @_;
my $plugin = MT->component('sample');
my $path = $plugin->get_config_value( 'filepath' );
$path = MT->config->FilePath if MT->config->FilePath;
( $path = $app->base ) =~ s!https?://!! unless $path;
$path = trim($path);
return unless ($path);
my $position = quotemeta(q{</body>});
my $plugin_tmpl = File::Spec->catdir( $plugin->path, 'tmpl', 'insert.tmpl' );
my $insert = qq{<mt:include name="$plugin_tmpl" component="sample" content="$path">\n};
$$tmpl =~ s/($position)/$insert$1/;
}
1;
変更点
- 11〜15行目
- 19行目:
content="$path"
入力値を取ってきます。
insert.tmpl
<!-- Add sample.js -->
<script type="text/javascript" src="<$mt:var name="content" escape="html"$>"></script>
<!-- Add sample.js -->
変更点
- 2行目:
<$mt:var name="content" escape="html"$>
動的になるように、変数に変更しました。
system.tmpl
<mtapp:setting
id="filepath"
label="<__trans phrase="FilePathLabel">"
show_hint="0"
hint="">
<input type="text" class="text col-10" name="filepath" id="filepath" value="<$mt:var name="filepath"$>" />
</mtapp:setting>
新規作成
insert.tmplと同じ階層に作成してください。
管理画面に設置する入力フィールドのhtmlを記載します。
CSS調整をしたい場合は、Bootstrapがデフォルトで使えます。
ここが冒頭でお話しした3ミリのフロントエンド要素です・・・
これで管理画面からファイルのパスを変更できるようになっているはずです!!!
おわりに
今回のプラグイン開発にあたり、参考にさせていただいた公式のドキュメントはこちらです。
ロジカルスタジオは、Movable Type開発元であるシックス・アパート株式会社の
パートナー企業制度「ProNet」へ加盟しております。
興味を持っていただけた方は、是非採用サイトよりご応募ください!